Creating a pop-out iframe with adoptNode and "magic iframes"
Update: 8th October - Significant issues with this doc. I caught up with Jake Archibald about this post because I thought I had something novel, during the conversation we uncovered a lot of things that make some of this post invalid, and I also learnt a lot in the process that I don't think most developers know. Calling .append() and .appendChild() adopt the node. This makes the usage of adoptNode in this instance useless because the append Algorithm ensures that the node is adopted. Read More
I lead the Chrome Developer Relations team at Google.
We want people to have the best experience possible on the web without having to install a native app or produce content in a walled garden.
Our team tries to make it easier for developers to build on the web by supporting every Chrome release, creating great content to support developers on web.dev, contributing to MDN, helping to improve browser compatibility, and some of the best developer tools like Lighthouse, Workbox, Squoosh to name just a few.
I love to learn about what you are building, and how I can help with Chrome or Web development in general, so if you want to chat with me directly, please feel free to book a consultation.
I'm trialing a newsletter, you can subscribe below (thank you!)
Meatspace Augmented Reality: From Chester to Nagoya
Some thoughts on AR after finding some during my travels. TL;DR - cheaper content creation and better discovery tools are needed. Read More
Photos from Carlisle Castle
I recently went on holiday with the boys and we swung past Carlisle Castle (birthplace of the world rebound Jake Archibald). It is one of the better castles that we've been to in the UK and I would heartily recommend spending some time visiting it if you are in the area. I didn't how significant the role was that Carlisle Castle played in the history of the England and Scotland, and it was great to find out more whilst we were there. Read More
Idle observation: Indexing text in images
I was out with the boys in Llangollen the other day (it's a beautiful town) and I was taking pictures of the informational signs containing some of the history of the area so that I could read it later, and I thought that I would look on the web to see if the information was available for more than just the people walking past the sign - and it's not. I then got thinking about the leaflets, the content in them is nearly impossible to find on the web. Read More
Liverpool World Museum
I took the kids to the Liverpool World History Museum the other week, it was pretty neat. The Space and Time section has not changed in about 30 years, a large section of the Bug enclosure was closed off, and the Aquarium seemed a tad smaller than I remember. The Egyptian section was open (it wasn't when I last went) and it was rather awesome. Read More
Bookstore - Llangollen
I love this place, it's on top of a cafe in Llangollen. I came here with my grandparents nearly 30 years ago and it's pretty much the same now. My only wish is that there was even more Commix books - I swear there was a heap more when I was a kid. Check it out Read More
Webmention.app — π
I love the idea of Webmentions, yet I've not had the time to implement it on my site. At a high-level web mentions let you comment, like and reply to other content on the web and have it be visible to that content without being centralised with tools like Disqus (which I am keen to remove from my site).
Web Mentions are split in to two components, the sender and the receiver. The receiver is the site that I am writing a post about and they might have something on their site that shows inbound links or reactions to their blog; and the sender is, well, me. I need to let the remote site that I have written or reacted to some content that they have created.
The rather awesome Remy Sharp created webmention.app to solve one part of the problem: sending pings. Remy's toolΒ makes it easy to send 'pings' to potential receivers that I have linked to, by simply calling a CLI script.
I host my blog using Zeit using Hugo and the static-builder tool, so it was relatively trivial for me to add in support for webmention app. I just npm i webmention
and then call the CLI version of the tool from my build.sh
file - it really is that simple.
Now when I create a post, it should send a quick ping to all new URL's that I have created some content about their site.
Creating a commit with multiple files to Github with JS on the web
My site is entirely static. It's built with Hugo and hosted with Zeit. I'm pretty happy with the setup, I get near instant builds and super fast CDN'd content delivery and I can do all the things that I need to because I don't have to manage any state. I've created a simple UI for this site and also my podcast creator that enables me to quickly post new content to my statically hosted site. Read More
Screen Recorder: recording microphone and the desktop audio at the same time — π
I have a goal of building the worlds simplest screen recording software and I've been slowly noodling around on the project for the last couple of months (I mean really slowly).
In previous posts I had got the screen recording and a voice overlay by futzing about with the streams from all the input sources. One area of frustration though was that I could not work out how to get the audio from the desktop and overlay the audio from the speaker. I finally worked out how to do it.
Firstly, getDisplayMedia
in Chrome now allows audio capture, there seems like an odd oversight in the Spec in that it did not allow you to specify audio: true
in the function call, now you can.
const audio = audioToggle.checked || false;
desktopStream = await navigator.mediaDevices.getDisplayMedia({ video:true, audio: audio });
Secondly, I had originally thought that by creating two tracks in the audio stream I would be able to get what I wanted, however I learnt that Chrome's MediaRecorder
API can only output one track, and 2nd, it wouldn't have worked anyway because tracks are like the DVD mutliple audio tracks in that only one can play at a time.
The solution is probably simple to a lot of people, but it was new to me: Use Web Audio.
It turns out that WebAudio API has createMediaStreamSource
and createMediaStreamDestination
, both of which are API's needed to solve the problem. The createMediaStreamSource
can take streams from my desktop audio and microphone, and by connecting the two together into the object created by createMediaStreamDestination
it gives me the ability to pipe this one stream into the MediaRecorder
API.
const mergeAudioStreams = (desktopStream, voiceStream) => {
const context = new AudioContext();
// Create a couple of sources
const source1 = context.createMediaStreamSource(desktopStream);
const source2 = context.createMediaStreamSource(voiceStream);
const destination = context.createMediaStreamDestination();
const desktopGain = context.createGain();
const voiceGain = context.createGain();
desktopGain.gain.value = 0.7;
voiceGain.gain.value = 0.7;
source1.connect(desktopGain).connect(destination);
// Connect source2
source2.connect(voiceGain).connect(destination);
return destination.stream.getAudioTracks();
};
Simples.
The full code can be found on my glitch, and the demo can be found here: https://screen-record-voice.glitch.me/
Extracting text from an image: Experiments with Shape Detection — π
I had a little down time after Google IO and I wanted to scratch a long-term itch I've had. I just want to be able to copy text that is held inside images in the browser. That is all. I think it would be a neat feature for everyone.
It's not easy to add functionality directly into Chrome, but I know I can take advantage of the intent system on Android and I can now do that with the Web (or at least Chrome on Android).
Two new additions to the web platform - Share Target Level 2 (or as I like to call it File Share) and the TextDetector
in the Shape Detection API - have allowed me to build a utility that I can Share images to and get the text held inside them.
The basic implementation is relatively straight forwards, you create a Share Target and a handler in the Service Worker, and then once you have the image that the user has shared you run the TextDetector
on it.
The Share Target API
allows your web application to be part of the native sharing sub-system, and in this case you can now register to handle all image/*
types by declaring it inside your Web App Manifest
as follows.
"share_target": {
"action": "/index.html",
"method": "POST",
"enctype": "multipart/form-data",
"params": {
"files": [
{
"name": "file",
"accept": ["image/*"]
}
]
}
}
When your PWA is installed then you will see it in all the places where you share images from as follows:
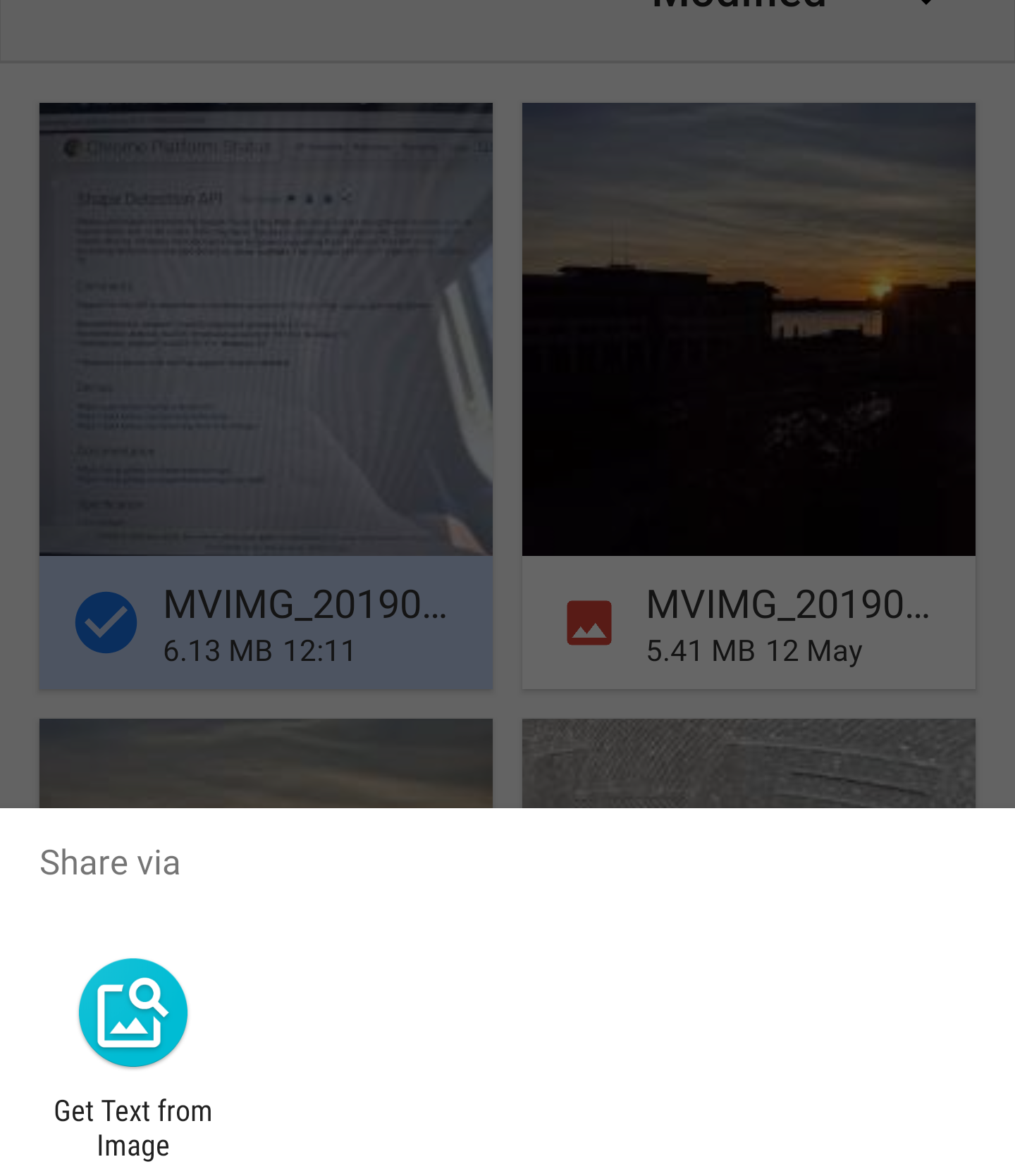
The Share Target
API treats sharing files like a form post. When the file is shared to the Web App the service worker is activated the fetch
handler is invoked with the file data. The data is now inside the Service Worker but I need it in the current window so that I can process it, the service knows which window invoked the request, so you can easily target the client and send it the data.
self.addEventListener('fetch', event => {
if (event.request.method === 'POST') {
event.respondWith(Response.redirect('/index.html'));
event.waitUntil(async function () {
const data = await event.request.formData();
const client = await self.clients.get(event.resultingClientId || event.clientId);
const file = data.get('file');
client.postMessage({ file, action: 'load-image' });
}());
return;
}
...
...
}
Once the image is in the user interface, I then process it with the text detection API.
navigator.serviceWorker.onmessage = (event) => {
const file = event.data.file;
const imgEl = document.getElementById('img');
const outputEl = document.getElementById('output');
const objUrl = URL.createObjectURL(file);
imgEl.src = objUrl;
imgEl.onload = () => {
const texts = await textDetector.detect(imgEl);
texts.forEach(text => {
const textEl = document.createElement('p');
textEl.textContent = text.rawValue;
outputEl.appendChild(textEl);
});
};
...
};
The biggest issue is that the browser doesn't naturally rotate the image (as you can see below), and the Shape Detection API needs the text to be in the correct reading orientation.
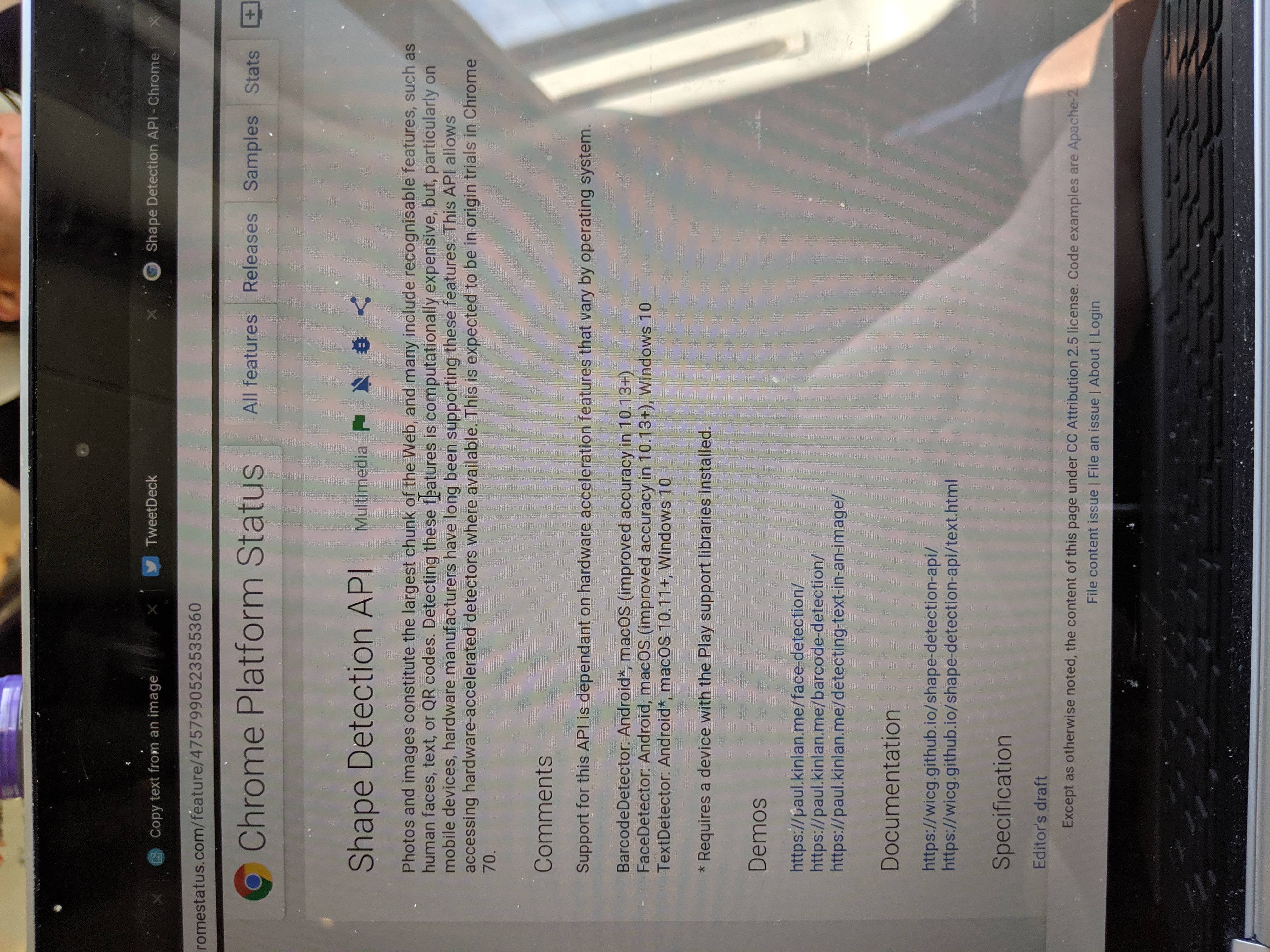
I used the rather easy to use EXIF-Js library to detect the rotation and then do some basic canvas manipulation to re-orientate the image.
EXIF.getData(imgEl, async function() {
// http://sylvana.net/jpegcrop/exif_orientation.html
const orientation = EXIF.getTag(this, 'Orientation');
const [width, height] = (orientation > 4)
? [ imgEl.naturalWidth, imgEl.naturalHeight ]
: [ imgEl.naturalHeight, imgEl.naturalWidth ];
canvas.width = width;
canvas.height = height;
const context = canvas.getContext('2d');
// We have to get the correct orientation for the image
// See also https://stackoverflow.com/questions/20600800/js-client-side-exif-orientation-rotate-and-mirror-jpeg-images
switch(orientation) {
case 2: context.transform(-1, 0, 0, 1, width, 0); break;
case 3: context.transform(-1, 0, 0, -1, width, height); break;
case 4: context.transform(1, 0, 0, -1, 0, height); break;
case 5: context.transform(0, 1, 1, 0, 0, 0); break;
case 6: context.transform(0, 1, -1, 0, height, 0); break;
case 7: context.transform(0, -1, -1, 0, height, width); break;
case 8: context.transform(0, -1, 1, 0, 0, width); break;
}
context.drawImage(imgEl, 0, 0);
}
And Voila, if you share an image to the app it will rotate the image and then analyse it returning the output of the text that it has found.
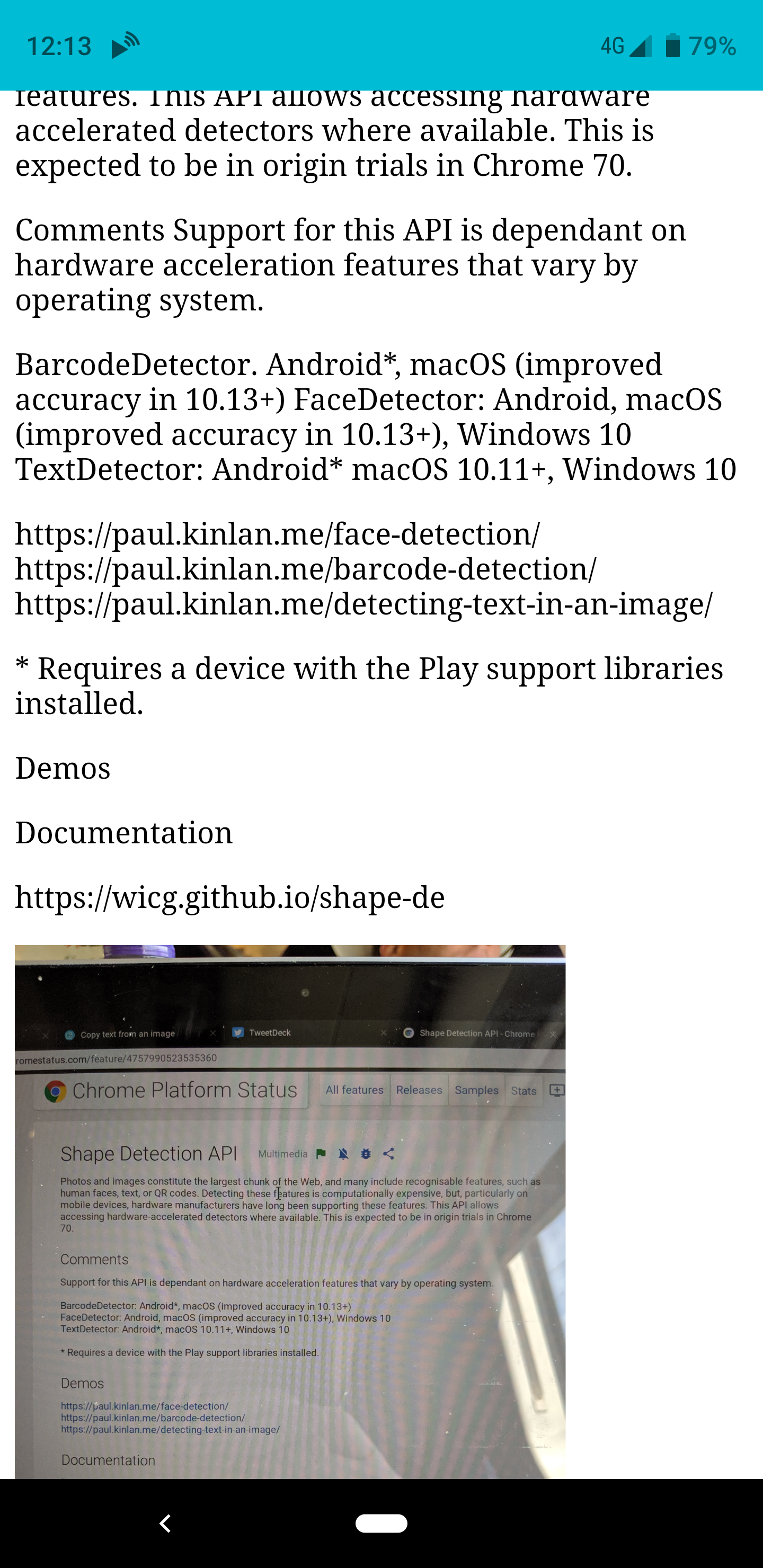
It was incredibly fun to create this little experiment, and it has been immediately useful for me. It does however, highlight the inconsistency of the web platform. These API's are not available in all browsers, they are not even available in all version of Chrome - this means that as I write this article Chrome OS, I can't use the app, but at the same time, when I can use it... OMG, so cool.
Small shrine in Engakuji Temple near Kamakura
Wood Carving found in Engakuji Shrine near Kamakura
Sakura
I'm told that more specifically that this is 'Yaezakura' Read More
Debugging Web Pages on the Nokia 8110 with KaiOS using Chrome OS
This post is a continuation of the post on debugging a KaiOS device with Web IDE, but instead of using macOS, you can now use Chrome OS (m75) with Crostini. I'm cribbing from the KaiOS Environment Setup which is a good start, but not enough for getting going with Chrome OS and Crostini. Below is the rough guide that I followed. Make sure that you are using at least Chrome OS m75 (currently dev channel as of April 15th), then: Read More
New WebKit Features in Safari 12.1 | WebKit — π
Big updates for the latest Safari!
I thought that this was a pretty huge announcement, and the opposite of Google which a while ago said that Google Pay Lib is the recommend way to implement payments... I mean, it's not a million miles away, Google Pay is built on top of Payment Request, but it's not PR first.
Payment Request is now the recommended way to pay implement Apple Pay on the web.
And my favourite feature given my history with Web Intents.
Web Share API
The Web Share API adds navigator.share(), a promise-based API developers can use to invoke a native sharing dialog provided the host operating system. This allows users to share text, links, and other content to an arbitrary destination of their choice, such as apps or contacts.
Now just to get Share Target API and we are on to a winner! :)
Offline fallback page with service worker — π
Years ago, I did some research into how native applications responded to a lack of network connectivity. Whilst I've lost the link to the analysis (I could swear it was on Google+), the overarching narrative was that many native applications are inextricably tied to the internet that they just straight up refuse to function. Sounds like a lot of web apps, the thing that set them apart from the web though is that the experience was still 'on-brand', Bart Simpson would tell you that you need to be online (for example), and yet for the vast majority of web experiences you get a 'Dino' (see chrome://dino).
We've been working on Service Worker for a long time now, and whilst we are seeing more and more sites have pages controlled by a Service Worker, the vast majority of sites don't even have a basic fallback experience when the network is not available.
I asked my good chum Jake if we have any guindance on how to build a generic fall-back page on the assumption that you don't want to create an entirely offline-first experience, and within 10 minutes he had created it. Check it out.
For brevity, I have pasted the code in below because it is only about 20 lines long. It caches the offline assets, and then for every fetch that is a 'navigation' fetch it will see if it errors (because of the network) and then render the offline page in place of the original content.
addEventListener('install', (event) => {
event.waitUntil(async function() {
const cache = await caches.open('static-v1');
await cache.addAll(['offline.html', 'styles.css']);
}());
});
// See https://developers.google.com/web/updates/2017/02/navigation-preload#activating_navigation_preload
addEventListener('activate', event => {
event.waitUntil(async function() {
// Feature-detect
if (self.registration.navigationPreload) {
// Enable navigation preloads!
await self.registration.navigationPreload.enable();
}
}());
});
addEventListener('fetch', (event) => {
const { request } = event;
// Always bypass for range requests, due to browser bugs
if (request.headers.has('range')) return;
event.respondWith(async function() {
// Try to get from the cache:
const cachedResponse = await caches.match(request);
if (cachedResponse) return cachedResponse;
try {
// See https://developers.google.com/web/updates/2017/02/navigation-preload#using_the_preloaded_response
const response = await event.preloadResponse;
if (response) return response;
// Otherwise, get from the network
return await fetch(request);
} catch (err) {
// If this was a navigation, show the offline page:
if (request.mode === 'navigate') {
return caches.match('offline.html');
}
// Otherwise throw
throw err;
}
}());
});
That is all. When the user is online they will see the default experience.
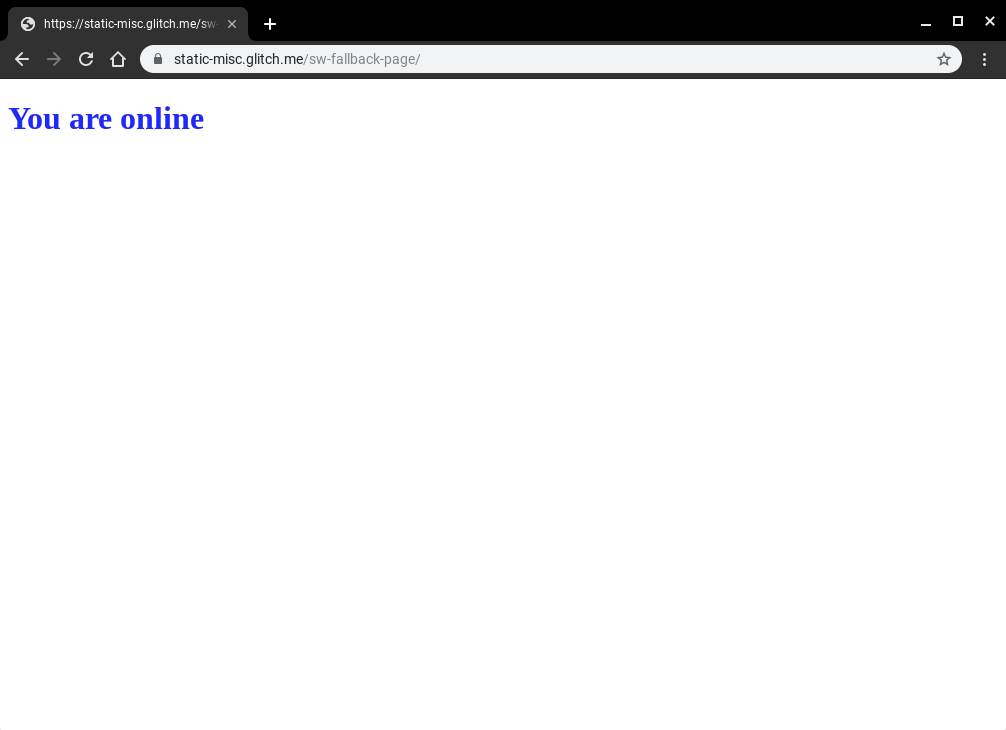
And when the user is offline, they will get the fallback page.
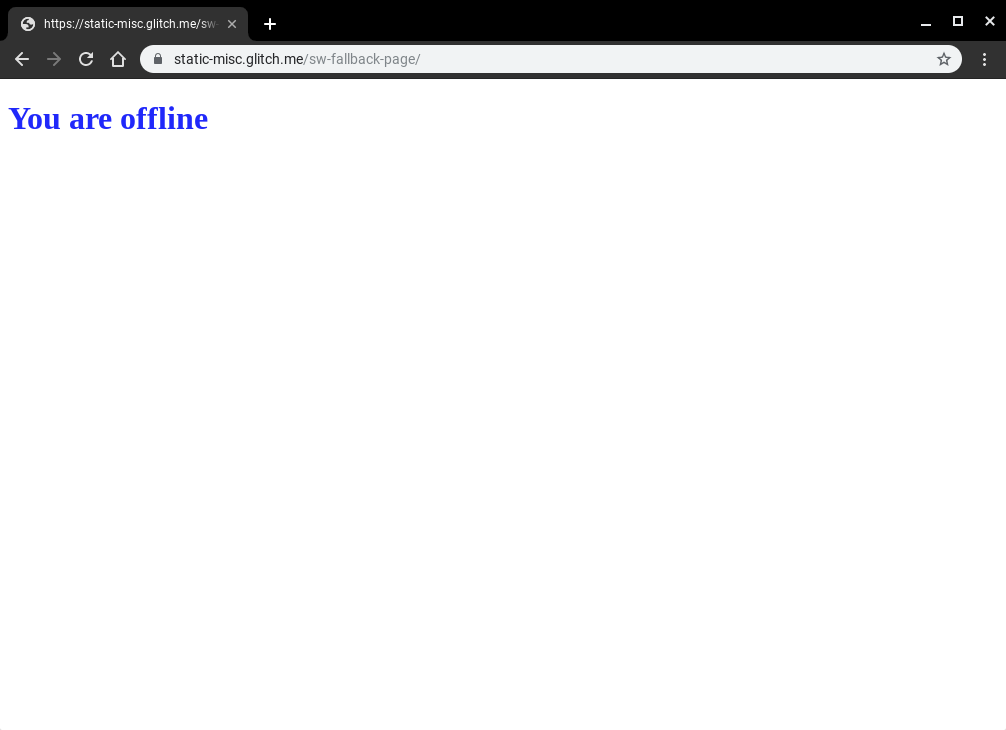
I find this simple script incredibly powerful, and yes, whilst it can still be improved, I do believe that even just a simple change in the way that we speak to our users when there is an issue with the network has the ability to fundamentally improve the perception of the web for users all across the globe.
Update Jeffrey Posnick kinldy reminded me about using Navigation Preload to not have to wait on SW boot for all requests, this is especially important if you are only controlling failed network requests.
testing block image upload
This is just a test to see if I got the image upload right. If you see this, then yes I did :) Read More
Editor.js — π
I've updated by Hugo based editor to try and use EditorJS as, well, the editor for the blog.
Workspace in classic editors is made of a single contenteditable element, used to create different HTML markups. Editor.js workspace consists of separate Blocks: paragraphs, headings, images, lists, quotes, etc. Each of them is an independent contenteditable element (or more complex structure) provided by Plugin and united by Editor's Core.
I think it works.
I struggled a little bit with the codebase, the examples all use ES Modules, however the NPM dist is all output in IIFE ES5 code. But once I got over that hurdle it has been quite easy to build a UI that looks a bit more like medium.
Quick Logcat - debugging android from the web — π
I was on the flight to Delhi this last week and I wanted to be able to debug my KaiOS device with Chrome OS - I never quite got to the level that I needed for a number of reasons (port forwarding didn't work - more on that in another post), but I did get to build a simple tool that really helps me build for the web on Android based devices.
I've been using WebADB.js for a couple of side projects, but I thought I would at least release one of the tools I made last week that will help you if you ever need to debug your Android device and you don't have adb
installed or any other Android system tools.
Quick LogCat is just that. It can connect to any Android device that is in developer mode and has USB enabled, is connected to your machine over USB and most importantly you grant access from the web page to connect to the device, and once that is all done it just runs adb shell logcat
to create the following output.
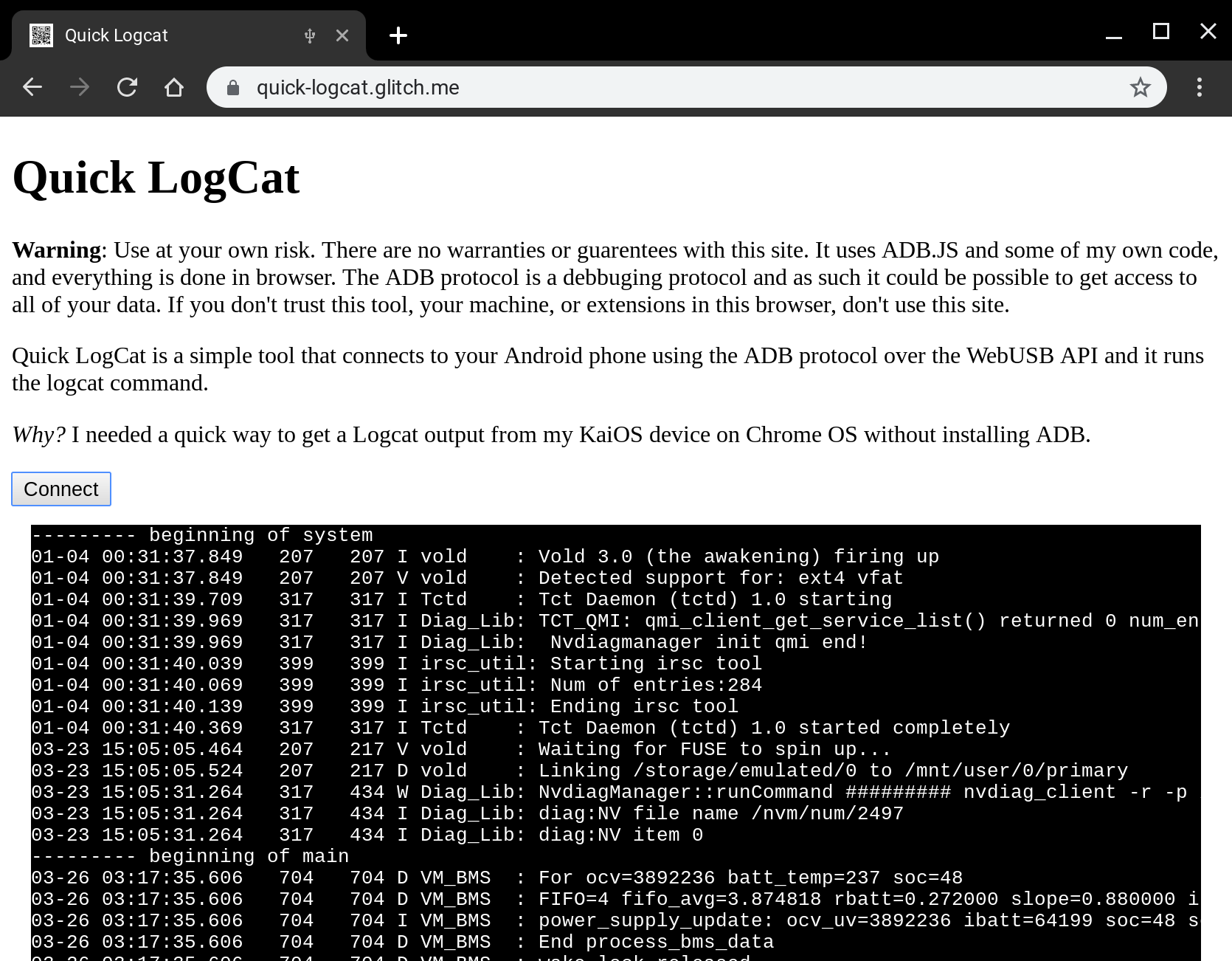
Checkout the source over on my github account, specifically the logger class that has the brunt of my logic - note a lot of this code is incredibly similar to the demo over at webadb.github.io, but it should hopefully be relatively clear to follow how I interface with the WebUSB API (which is very cool). The result is the following code that is in my index file: I instantiate a controller, connect to the device which will open up the USB port and then I start the logcat
process and well, cat
the log, via logcat
.
It even uses .mjs files :D
<script type="module">
import LogcatController from "/scripts/main.mjs";
onload = () => {
const connect = document.getElementById("connect");
const output = document.getElementById("output");
let controller = new LogcatController();
connect.addEventListener("click", async () => {
await controller.connect();
controller.logcat((log) => {
output.innerText += log;
})
});
};
</script>
ADB is an incredibly powerful protocol, you can read system files, you can write over personal data and you can even easily side-load apps, so if you give access to any external site to your Android device, you need to completely trust the operator of the site.
This demo shows the power and capability of the WebUSB API, we can interface with hardware without any natively installed components, drivers or software and with a pervasive explicit user opt-in model that stops drive-by access to USB components.
I've got a couple more ideas up my sleeve, it will totally be possible to do firmware updates via the web if you so choose. One thing we saw a lot of in India was the ability side-load APK's on to user's new phones, whilst I am not saying we must do it, a clean web-interface would be more more preferable to the software people use today.
What do you think you could build with Web USB
and adb
access?
Debugging Web Pages on the Nokia 8110 with KaiOS
We've been doing a lot of development on feature phones recently and it's been hard, but fun. The hardest bit is that on KaiOS we found it impossible to debug web pages, especially on the hardware that we had (The Nokia 8110). The Nokia is a great device, it's built with KaiOS which we know is based on something akin to Firefox 48, but it's locked down, there is no traditional developer mode like you get on other Android devices, which means you can't connect Firefox's WebIDE easily. Read More