I love Puppeteer - it lets me play around with the ideas of The Headless Web - that is running the web in a browser without a visible browser and even build tools like DOM-curl (Curl that runs JavaScript). Specifically I love scripting the browser to scrape, manipulate and interact with pages.
One demo I wanted to make was inspired by Ire's Capturing 422 live images post where she ran a puppeteer script that would navigate to many pages and take a screenshot. Instead of going to many pages, I wanted to take many screenshots of elements on the page.
The problem that I have with Puppeteer is the opening stanza that you need to do anything. Launch, Open tab, navigate - it's not complex, it's just more boilerplate than I want to create for simple scripts. That's why I created Puppeteer Go. It's just a small script that helps me build CLI utilities easily that opens the browser, navigates to a page, performs your action and then cleans up after itself.
Check it out.
const { go } = require('puppeteer-go');
go('https://paul.kinlan.me', async (page) => {
const elements = await page.$$("h1");
let count = 0;
for(let element of elements) {
try {
await element.screenshot({ path: `${count++}.png`});
} catch (err) {
console.log(count, err);
}
}
});
The above code will find the h1 element in my blog and take a screenshot. This is nowhere near as good as Ire's work, but I thought it was neat to see if we can quickly pull screenshots from canisuse.com directly from the page.
const { go } = require('puppeteer-go');
go('https://caniuse.com/#search=css', async (page) => {
const elements = await page.$$("article.feature-block.feature-block--feature");
let count = 0;
for(let element of elements) {
try {
await element.screenshot({ path: `${count++}.png`});
} catch (err) {
console.log(count, err);
}
}
});
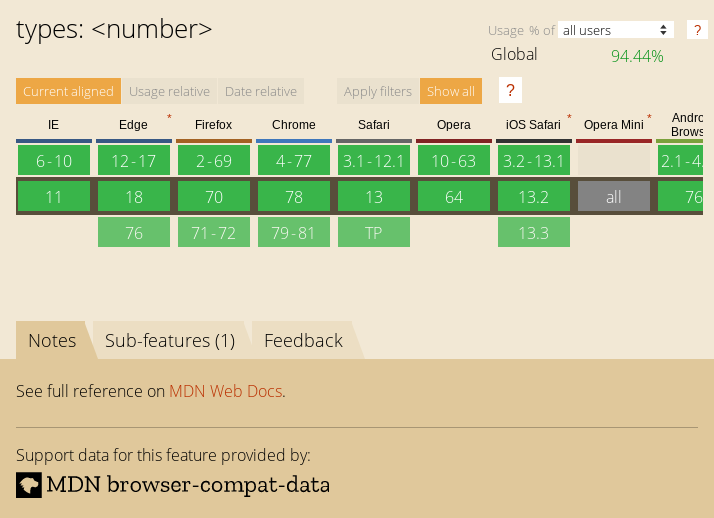
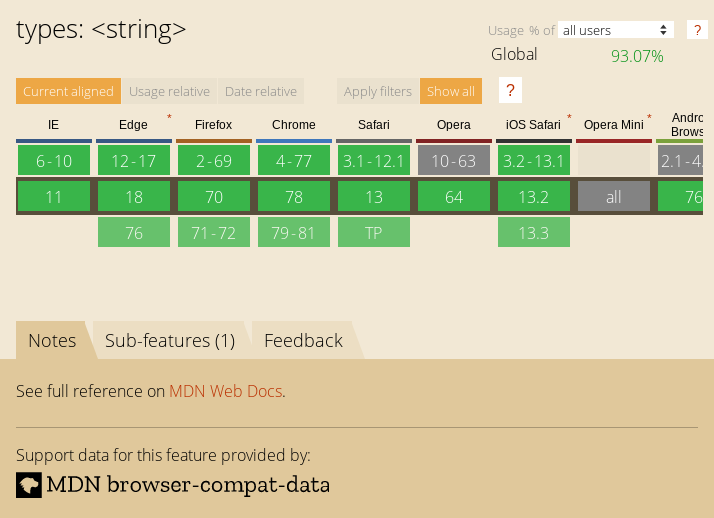
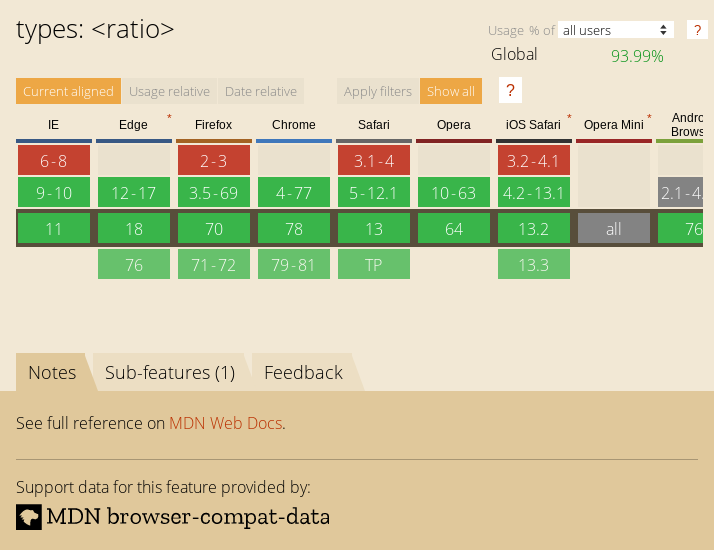
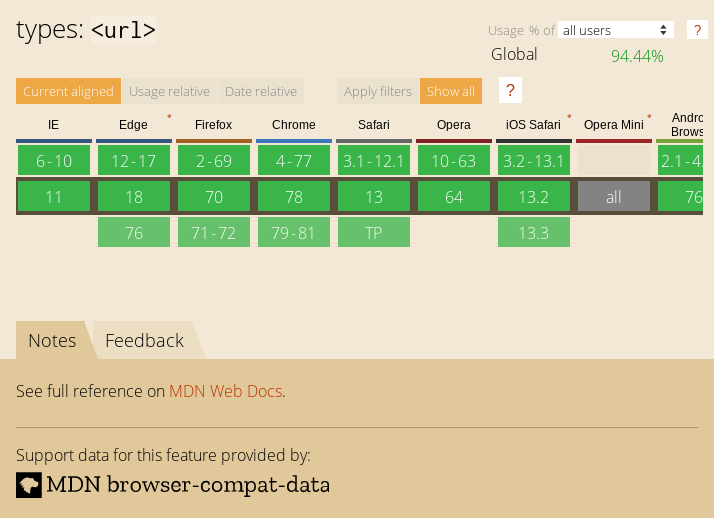
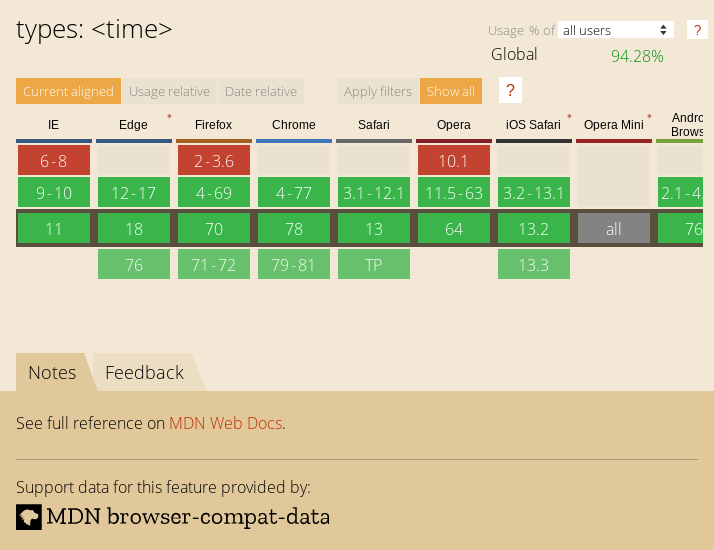
Enjoy!
I lead the Chrome Developer Relations team at Google.
We want people to have the best experience possible on the web without having to install a native app or produce content in a walled garden.
Our team tries to make it easier for developers to build on the web by supporting every Chrome release, creating great content to support developers on web.dev, contributing to MDN, helping to improve browser compatibility, and some of the best developer tools like Lighthouse, Workbox, Squoosh to name just a few.
I love to learn about what you are building, and how I can help with Chrome or Web development in general, so if you want to chat with me directly, please feel free to book a consultation.
I'm trialing a newsletter, you can subscribe below (thank you!)